Hosted checkout form
This is the simplest and most popular approach. From your pricing page, a customer can click a “pay button” and be directed to our hosted checkout form - we will handle everything from there.
This is the simplest and most popular approach. From your pricing page, a customer can click a “pay button” and be directed to our hosted checkout form - we will handle everything from there:
-
The form can be fully customized to fit your branding
-
All of your enabled payment options (ex: credit card/apple pay/ACH/crypto/etc.) will be shown
-
You will receive the appropriate webhook events on success/failure
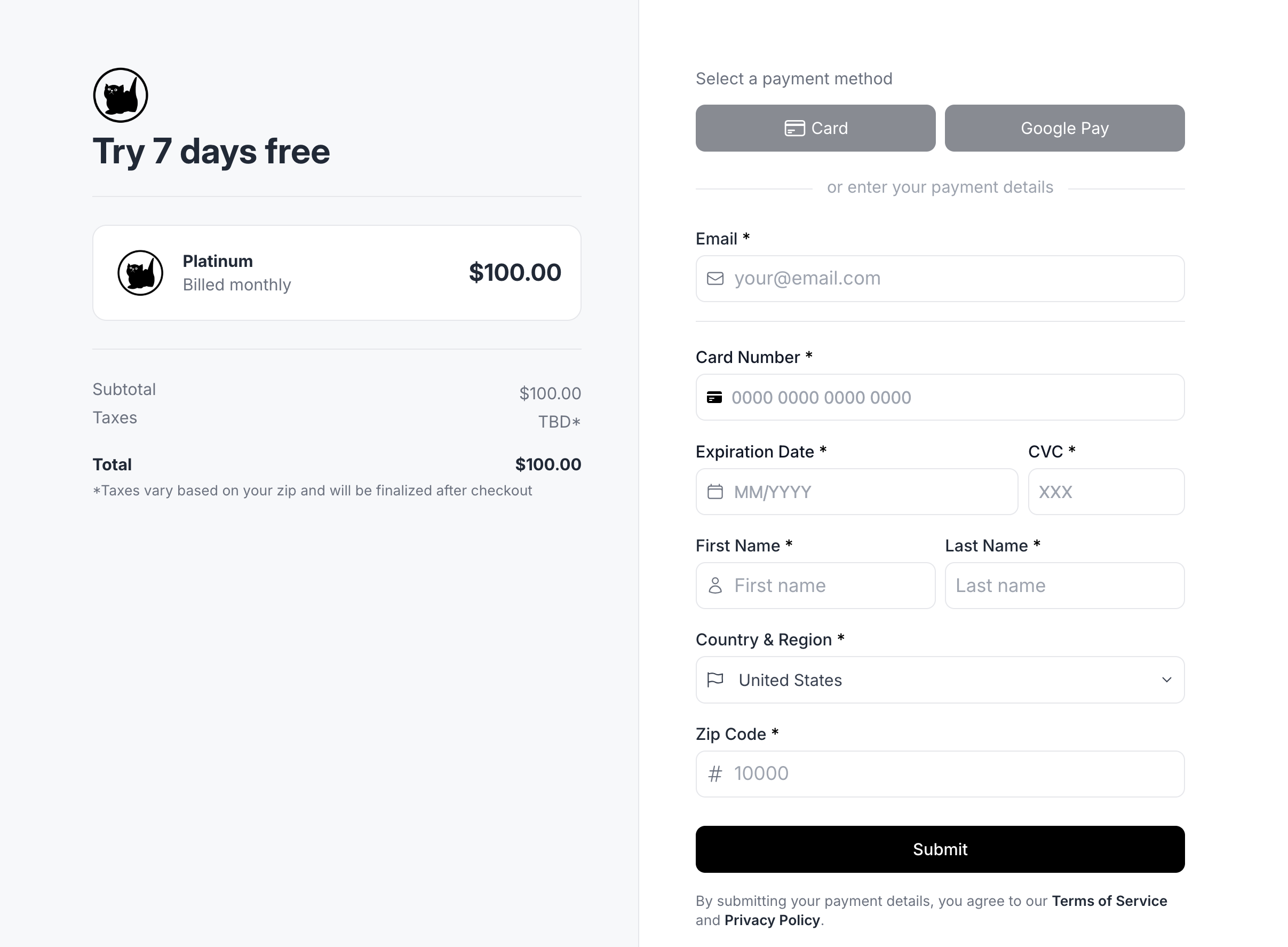
Once a customer has submitted the checkout form, this will automatically sync into our system and you will see the customer in your OpenPay admin console with the subscription or one-time price that they purchased.
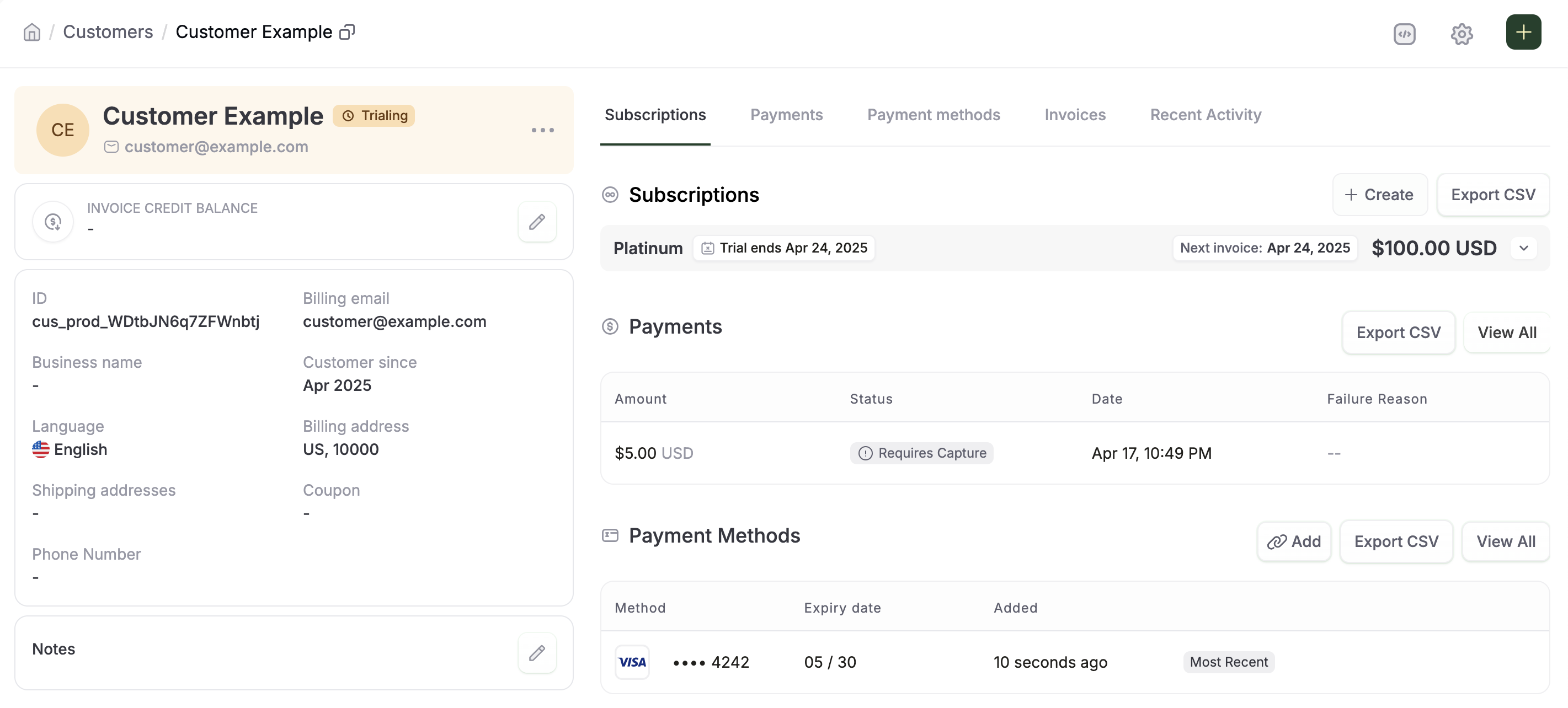
Install and import our clientCopied!
For this recipe, we will use the Python SDK, which you can install using pip install getopenpay
or poetry add getopenpay
from getopenpay.client import ApiKeys, OpenPayClient
OP_PUBLISHABLE_TOKEN = 'TODO_YOUR_PUBLISHABLE_TOKEN'
OP_SECRET_TOKEN = 'TODO_YOUR_SECRET_TOKEN'
api_keys = ApiKeys(publishable_key=OP_PUBLISHABLE_TOKEN, secret_key=OP_SECRET_TOKEN)
# sandbox/staging environment
sandbox_host = 'https://connto.openpaystaging.com'
# production environment
production_host = 'https://connto.getopenpay.com'
client = OpenPayClient(api_keys=api_keys, host=sandbox_host)
Create customerCopied!
create_customer_request = CreateCustomerRequest(
email='test_customer+1@getopenpay.com',
first_name='John',
last_name='Smith',
line1='123 Main St',
city='San Francisco',
state='CA',
country='US',
zip_code='94105'
)
customer_external = op_client.customers.create_customer(
create_customer_request=create_customer_request.to_dict()
)
Create a checkout sessionCopied!
checkout = op_client.checkout.create_checkout_session(
CreateCheckoutSessionRequest(
customer_id=customer_id, # <- pass in cutomser_id
line_items=[
CreateCheckoutLineItem(price_id='TODO_DESIRED_PRICE_1', quantity=2),
CreateCheckoutLineItem(price_id='TODO_DESIRED_PRICE_2', quantity=3),
],
# subscriptions are created for this user on checkout:
mode=CheckoutMode.SUBSCRIPTION,
return_url='https://your_return_url_after_checkout',
success_url='https://your_success_url_after_checkout',
)
)
Reroute to our hosted pageCopied!
print(f'Reroute customer to this page for payment: {checkout_session.url})