Create subscription
Experience the ease of creating subscriptions with OpenPay's intuitive design and robust features.
Subscriptions grant customers access to your products or services for a set duration. Using OpenPay, you can streamline recurring billing, offer trial periods, and tailor subscription options to meet the specific needs of each customer.
There are a variety of ways you can create subscriptions in OpenPay.
In the admin console, you can navigate to the subscriptions tab and click [+ Add Subscription] on the top right to open the subscription creation workflow.
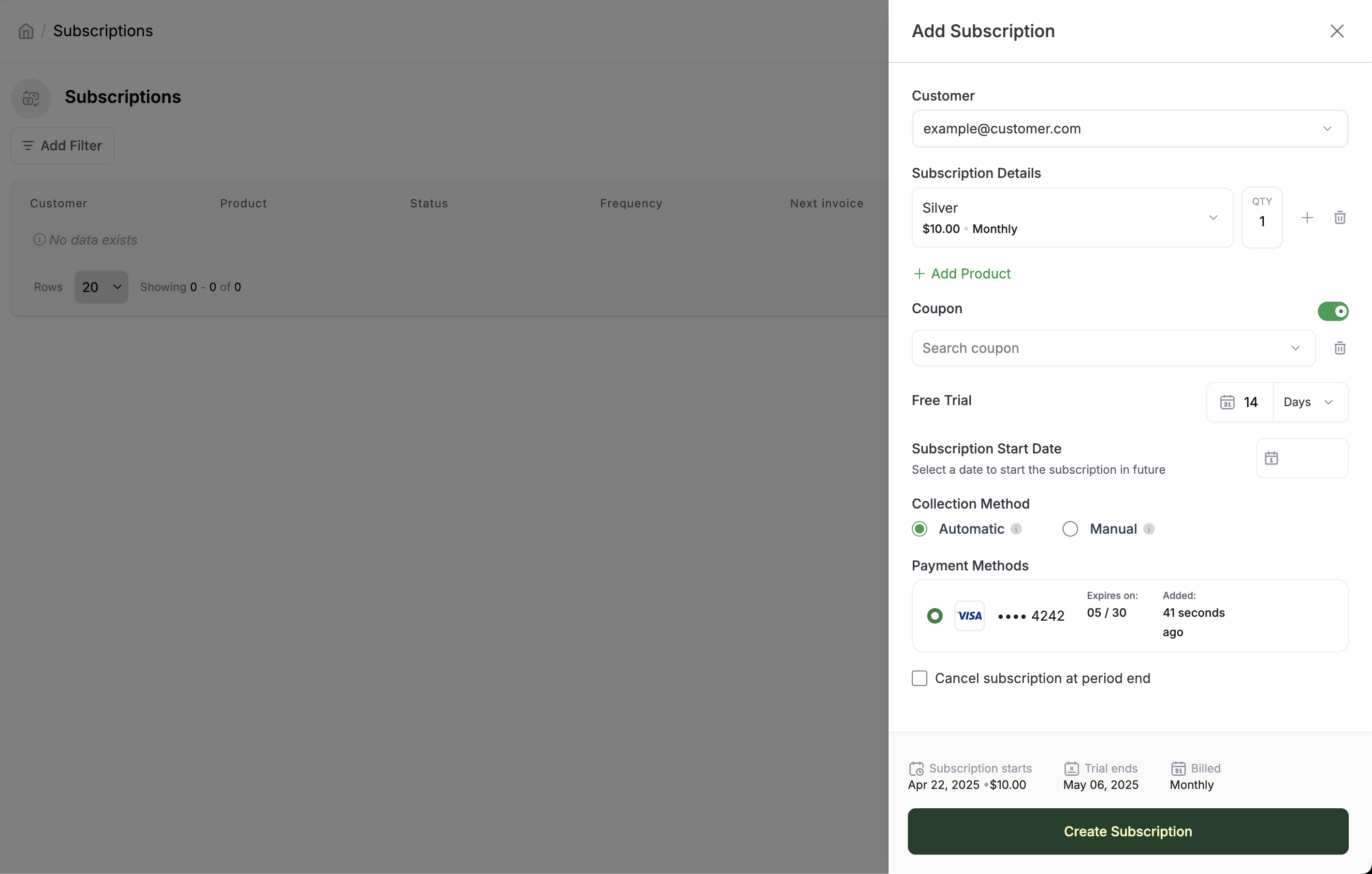
You can also use one of our shortcuts to save yourself the extra step.
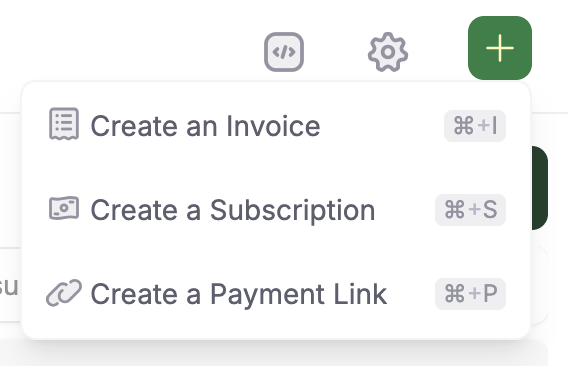
See how you can include add-ons in a subscription here:
Add-onsAdd-ons are supplementary charges applied on top of a subscription's main price.
Create link via admin consoleCopied!
Payment linksEasily create a fully functional payment page in just a few seconds and share the link with your customers—no code necessary.
Create link via APICopied!
OpenPay.jsCopied!
Setup payment method and create subscriptionsA step-by-step guide for setting up payment methods and creating subscriptions using the OpenPay SDK and OpenPay.js
OpenPay ReactCopied!
Setup payment method and create subscriptionsA step-by-step guide for setting up payment methods and creating subscriptions using the OpenPay SDK and OpenPay.js
Install and import the OpenPay SDKCopied!
For this recipe, we will use the Python SDK, which you can install using pip install getopenpay
or poetry add getopenpay
.
from openapi_client.models import (
CalendarIntervalEnum,
CreateCustomerRequest,
CreatePriceRequest,
CreateProductRequest,
CreateSubscriptionRequest,
PriceTypeEnum,
PricingModel,
SelectedPriceQuantity
)
from openpay.client import ApiKeys, OpenPayClient
Initialize the SDK with your API keysCopied!
You will need both a Publishable Key and a Secret Key. If you don't have such a pair of keys yet, follow this guide.
client = OpenPayClient(
api_keys=ApiKeys(
publishable_key='TODO_YOUR_PUBLISHABLE_KEY',
secret_key='TODO_YOUR_SECRET_KEY'
)
)
Create a productCopied!
The Product object is the foundation of every subscription. It is a representation of the service you want to offer on your platform.
Define the attributes of your product. You can link it to an SKU in your existing system, list the features that differentiate this product from the others, and even choose what each unit of this product should be called.
product = client.products.create_product(
CreateProductRequest(
name='Standard license',
description='For personal use',
account_sku='970000000001',
features=['Priority support'],
unit_label='seat',
is_active=True
)
)
Create a priceCopied!
Define the value of your Product with a Price object. Our API gives you the ability to create prices as simple or as complex as you want, from basic flat-rate models to tiered models that charge based on usage.
price_amount_atom = 9000 # = $90.00
price = client.prices.create_price_for_product(
CreatePriceRequest(
product_id=product.id,
unit_amount_atom=price_amount_atom,
is_active=True,
# Simple pricing model with a flat rate and 7-day trial
pricing_model=PricingModel.STANDARD,
trial_period_days=7,
# Bill every month
price_type=PriceTypeEnum.RECURRING,
billing_interval=CalendarIntervalEnum.MONTH,
billing_interval_count=1,
# Require a minimum of two months' commitment
contract_term_multiple=2,
contract_auto_renew=True,
)
)
Create a customerCopied!
Now that you have a product with a price defined for it, create a customer who will be subscribing to it.
customer = client.customers.create_customer(
CreateCustomerRequest(
first_name='John',
last_name='Doe',
line1='123 Main St',
city='Anytown',
state='Anystate',
country='US',
zip_code='12345',
email='john.doe@example.com'
)
)
Create a subscriptionCopied!
Assuming your customer's already attached a payment method to their account, you can now create a subscription for the price you just made with that payment method as the default.
If all goes well, you should now have a subscription marked active
!
subscription = client.subscriptions.create_subscription(
CreateSubscriptionRequest(
customer_id=customer.id,
payment_method_id='TODO_PAYMENT_METHOD_ID',
selected_product_price_quantity=[
SelectedPriceQuantity(price_id=price.id, quantity=1)
],
)
)
print(subscription.status)